Finding Nemo - Implementing Xamarin.Forms SearchBar
Xamarin.Forms gives you a component called the SearchBar (documentation), by default. In this post I will show you what it can do and how to use it.
Like you would expect, this gives you a simple text edit box which is specifically designed to serve in search operations.
To show you how easy it is to implement, I will demonstrate how I built it into the sample app I have been using up till now, if you need to refresh your memory take a look at my previous post. In this app there is an employee directory. Although this isn’t a endless list, it is desirable to quickly navigate to the right person. And how could we do it better than by searching?
First let’s take a look at how my ListView is before I make any changes.
<ListView.ItemTemplate>
<ImageCell.ContextActions>
<MenuItem Text="Stuur e-mail" Clicked="EmployeeContextEmail\_OnClicked"></MenuItem>
</ImageCell.ContextActions>
</ImageCell>
</DataTemplate>
</ListView.ItemTemplate>
</ListView>
As you can see I’ve made use of a simple StackLayout to put in a ListView which can be pulled to refresh, does something when an item is tapped and tells the list how to display a cell to the user.
In the running app this will be shown as underneath.
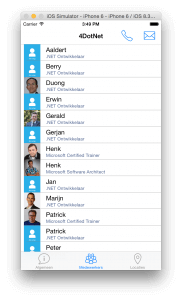
Adding a SearchBar is pretty easy, just go like this:
[…] […]
The Placeholder attribute sets the placeholder text when no search-term hasn’t been entered. The TextChanged is as advertised on the tin; the event that gets fired when the text is changed.
For the end-user the screen now looks like this;
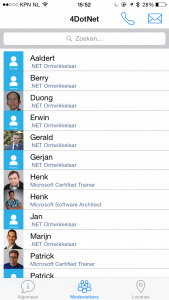
Now lets have a look at the SearchBar_TextChanged event-handler.
private void SearchBar_OnTextChanged(object sender, TextChangedEventArgs e) { EmployeeListView.BeginRefresh();
if (string.IsNullOrWhiteSpace(e.NewTextValue)) EmployeeListView.ItemsSource = _container.Employees; else EmployeeListView.ItemsSource = _container.Employees.Where(i => i.Name.Contains(e.NewTextValue));
EmployeeListView.EndRefresh(); }
We are starting with telling the ListView that we are going to do some updates, so it will probably skip some redraw operations while we update the data inside it.
After that we check if the user has entered text, or cleared all text from the SearchBar and supply a corresponding ItemSource. Don’t forget to call the EndRefresh method, and that’s about it! Simple is it not?!
Now when you start entering text in the field it will start filtering in your ListView.
As a next step you would want to clean up your form code and not handle this there. So create a inheritance of a ListView which will handle filtering in its own, or create a composition of the SearchBar and the ListView to form a whole new component, a component which I like to call..
So far on the SearchBar for right now! Hope you’ll put it to good use.