OTP Auto-Fill Entry for iOS with Xamarin.Forms
I have to admit, after reading the tweet below I assumed it was about iOS, but of course this should have also been about Android. Anyway, I implemented the iOS APIs on how to catch that One-Time Password (OTP) that you might receive through a text and let it be autofilled in your Entry.
Is possible to automatically read an OTP SMS during signup with #Xamarin ? @xamarinhq @XamExpertDay @jfversluis
— Josiah Mahachi 🇿🇼 (@jmahachi) October 13, 2020
TL;DR, find the code here: https://github.com/jfversluis/XFiOSOTPEntrySample look for a custom renderer in the iOS project.
What Is This OTP AutoFill? #
If you are using iOS or a developer developing for iOS, you might have seen the functionality where you have two-factor authentication (2FA) set up, you receive a text and the iOS keyboard will suggest that you paste that code in the text field that is focussed at that time.
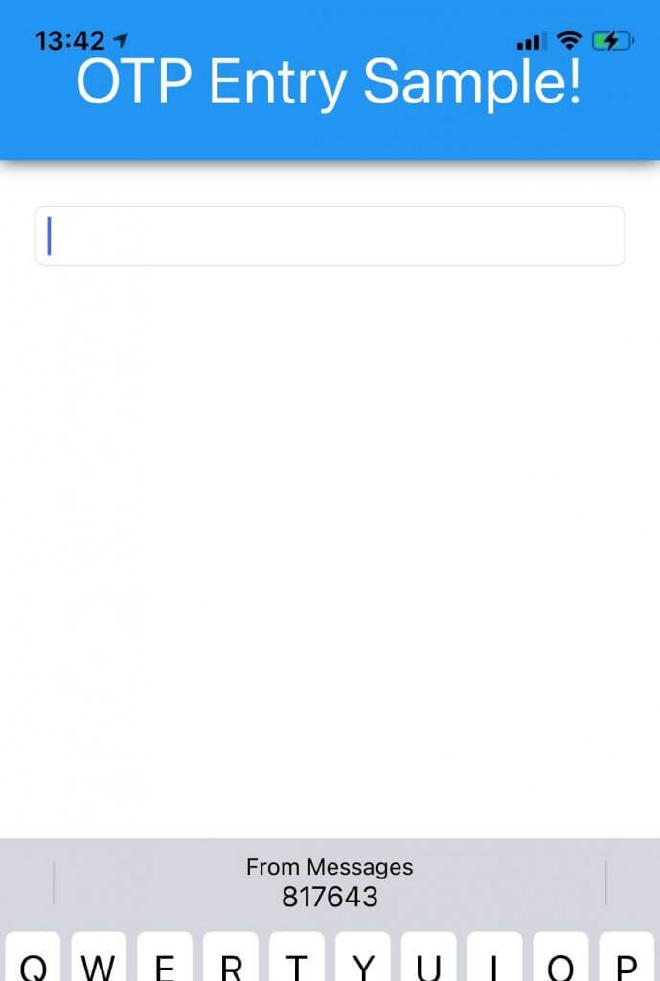
This is actually one line to enable this in your iOS code, but for Xamarin.Forms you have to dig a bit deeper.
Enable The OTP Functionality with a Custom Renderer #
We’ve already learned how to create custom renderers in
this blog and this video (subscribe!), so I won’t go too much into that. What I implemented is custom control, the OtpEntry
which is simply an inheritance of Entry, nothing fancy about that. But that helps me to apply the OTP functionality to _only_ that entry type instead of _all_ entries.
Then the renderer code looks like below.
Note that there is a check there to see if the iOS version is iOS 12+. That is the version where this functionality was implemented.
From there, we set the TextContentType
property to a UITextContentType.OneTimeCode
and that is all is needed to enable this. Not use your entry in a page, something like <iosotpentrysample:OtpEntry Margin="20" />
and whenever you receive a text, the keyboard should pop up with that code. Actually, that was a fun thing to figure out; testing this.
Testing the Code #
I think Apple opted at some point to standardize the two-factor authentication text messages so they are easily parsable and can be used for these types of scenarios. However, that did not happen (yet), so how does it know when a code comes in?
To be honest, pure magic. I don’t know. There is also no way built-in the Simulator to fake a text coming in, so you can only test this on your physical device. I tried to text myself a simple code like 123456. That didn’t work.
Then I thought: let’s just look at a message I got from a service that sends these actual messages. So I looked in my inbox and found one that said: “Your security code is 123456”. I simply tried to add that text and a random code… And voila! That worked :D
So text yourself something like that and switch back to your app to test it. There is no rush, the code should be shown for 3 minutes before it stops suggesting it
All the code can be found here for your reference.