Get more reviews for your Xamarin.Forms app with iOS 10.3
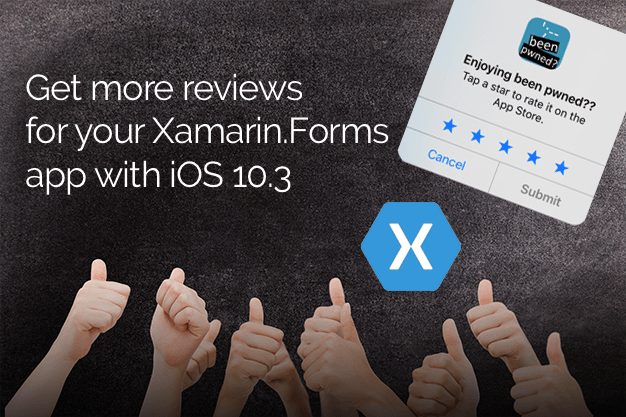
With the introduction of iOS 10.3 a small, but rather useful feature was added. It’s called the SKStoreReviewController. This addition to the iOS framework can boost the number of reviews that you gather for your app. When you have released your app, it is always nice to get some recognition for it in the form of the much desired 5-star ratings.
Rating then #
Until now, this has been a rather painful process, especially for iOS. To trigger a user to leave a review or rating for your app there have been three URLs that you should be using.
For iOS 7 and below there was this one:
itms-apps://itunes.apple.com/WebObjects/MZStore.woa/wa/viewContentsUserReviews?type=Purple+Software&id=APP_ID
As of iOS 7 up to 8 you could use:
itms-apps://itunes.apple.com/app/idAPP_ID (that is right, id is directly attached to your APP_ID)
And from iOS 8 you could use:
itms-apps://itunes.apple.com/WebObjects/MZStore.woa/wa/viewContentsUserReviews?id=APP_ID&onlyLatestVersion=true&pageNumber=0&sortOrdering=1&type=Purple+Software
And none of these take you directly to a page where the user can start writing their review. For this they have later added the ?action=write-review suffix for the URLs.
Rating now #
To overcome this, a lot of app developers have been building their own mechanism to seduce users to leave a rating. Mostly this is done by showing a popup which is shown far too often for my taste. To provide for a more consistent, in-app experience for the user, Apple has now introduced the SKStoreReviewController functionality.
Note: this feature cannot be triggered manually. This pop-up will be shown at the discretion of Apple. They have implemented logic to determine how often and when the pop-up is shown. You cannot use this to prompt the user for a review or rating whenever you want. It seems the pop-up will come up 3-4 times a year. It will always show when debugging so you can test it, but it will never come up when distributed through TestFlight.
The code #
To implement this you actually just need one line;
if(UIDevice.CurrentDevice.CheckSystemVersion(10, 3)) { SKStoreReviewController.RequestReview(); }
OK, 4 lines counting everything. Because this feature was introduced recently, as of iOS 10.3, you will need to see if the device is running that version.
If we were to implement this in a Xamarin.Forms app, you will need to use the DependencyService. In our shared code we would introduce a simple interface, like so:
public interface IRatingService { void ShowRatingPrompt(); }
Because this is a iOS specific feature, we only need the iOS implementation. So in your iOS project, implement this interface:
[assembly: Xamarin.Forms.Dependency (typeof (RatingService_iOS))]
namespace MyApp { public class RatingService_iOS { public void ShowRatingPrompt() { if(UIDevice.CurrentDevice.CheckSystemVersion(10, 3)) { SKStoreReviewController.RequestReview(); } } } }
And finally, from our shared code we can now invoke this by simply adding this:
if (Device.RuntimePlatform == Device.iOS) DependencyService.Get ()?.ShowRatingPrompt ();
You probably want to do this somewhere when the app is launched. Because Apple is in charge of if and when the pop-up is shown, you do not need to worry about that yourself. Just remember to only execute this code on iOS.
I have implemented it in my have i been pwned app for iOS, and then it looks like this:
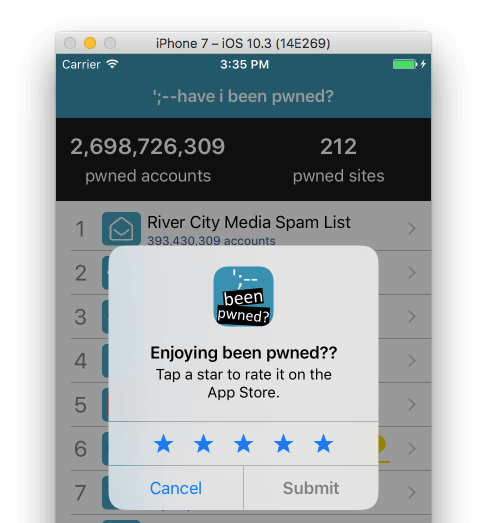
So what about Android? As far as I know they do not have this kind of functionality out of the box. To trigger a review manually they do have a URL available for you; https://play.google.com/store/apps/details?id=YOUR_APP_ID or at least, that takes them to the right page. For a periodic pop-up to request user feedback you will still need some custom logic or a third-party plugin.